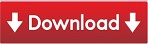
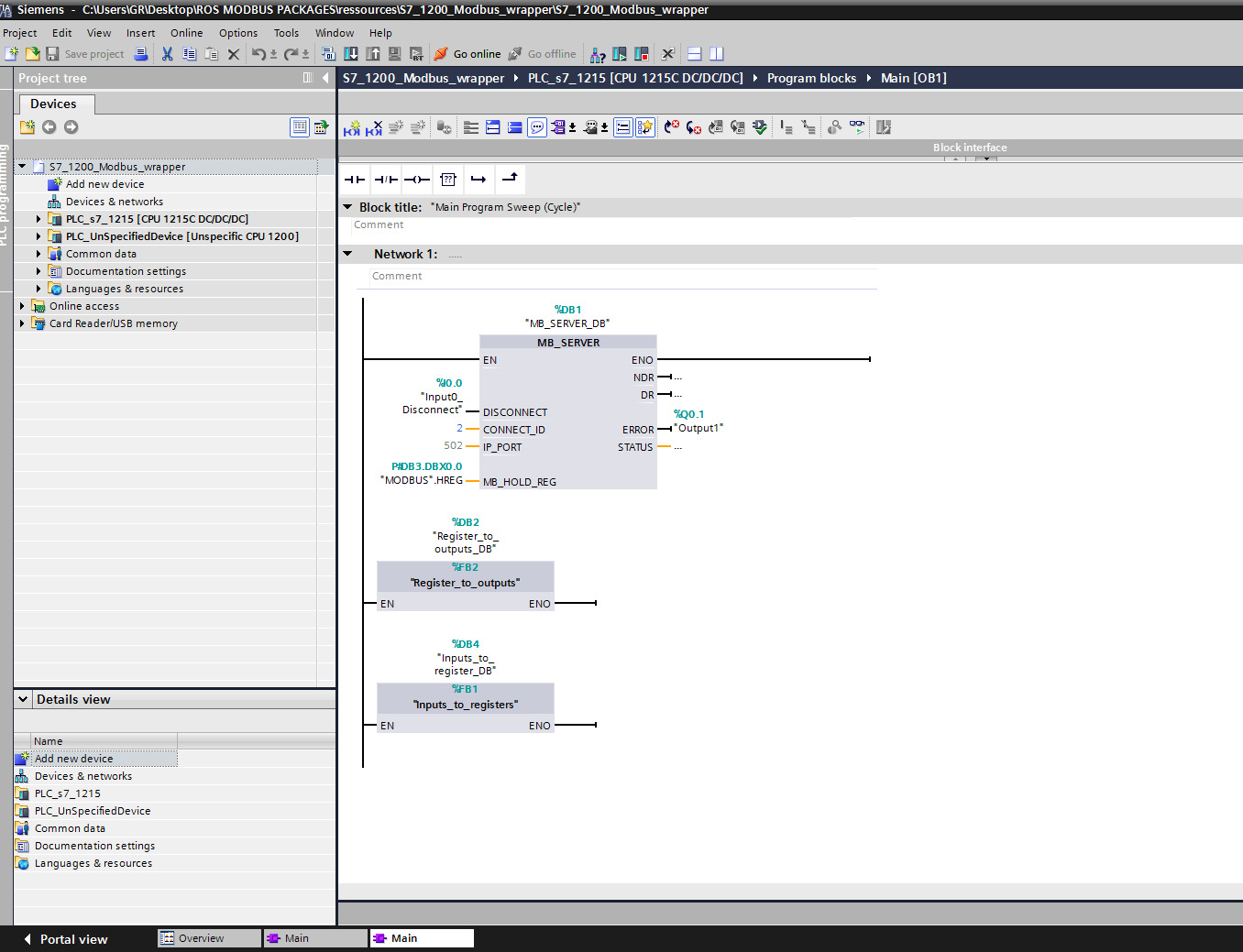
In order to test this out I'll just create a simple TCP server with pymodbus and docker. To do so I turn to three main libraries: pymodbusm threading and flask (and flask-socketio). But sometimes I just want something quick to figure out if the sensor is putting formation out that I can check form a website.
#Python modbus server software#
There are several libraries and software capable of pulling all the data, ranging from a celery systems to a kafka implementation. You can use a variety of software to present this information, however most of the time you are limited to just reading the registers with no additional transformations applied to the information. Quite often I find the need to have a simple display for showing the data coming from a modbus TCP interface. sleep ( 0.01 ) open_tool () back_to_observation () # Activate learning mode and close connexion client. read_holding_registers ( 150, count = 1 ). write_register ( 100, 1 ) # Wait for end of Move command while client. write_registers ( 0, joints_to_send ) client. value shape_picked, color_picked = vision_pick ( workspace_name, height_offset, shape, color ) # - Go to release pose joints = joints_to_send = list ( map ( lambda j : int ( number_to_raw_data ( j * 1000 )), joints )) client. write_registers ( 500, 1 ) print 'VISION PICK - pick a red pawn, lift it and release it' shape = ShapeEnum.
#Python modbus server update#
pose auto_calibration () back_to_observation () # update tool client. connect () print "Connected to modbus server" # launch auto calibration then go to obs. registers ) return result_shape_int, result_color_int # - Main programm if _name_ = '_main_' : print "- START" client = ModbusTcpClient ( 'localhost', port = 5020 ) # - Variable definition # To change workspace_name = 'gazebo_1' height_offset = 0.0 # connect to modbus server client. registers ) result_color_int = raw_data_to_number ( client. sleep ( 0.01 ) # - Check result : SHAPE AND COLOR result_shape_int = raw_data_to_number ( client. write_registers ( 612, 1 ) # Wait for end of function while client. write_registers ( 625, number_to_raw_data ( color_int )) # launch vision pick function client. write_registers ( 624, number_to_raw_data ( shape_int )) client. sleep ( 0.05 ) # Function to call Modbus Server vision pick function def vision_pick ( workspace_str, height_offset, shape_int, color_int ): register_workspace_name ( workspace_str ) register_height_offset ( height_offset ) client. write_register ( 513, 1 ) else : client. registers def open_tool (): tool_id = get_current_tool_id () if tool_id = 31 : client. read_input_registers ( 200, count = 1 ).
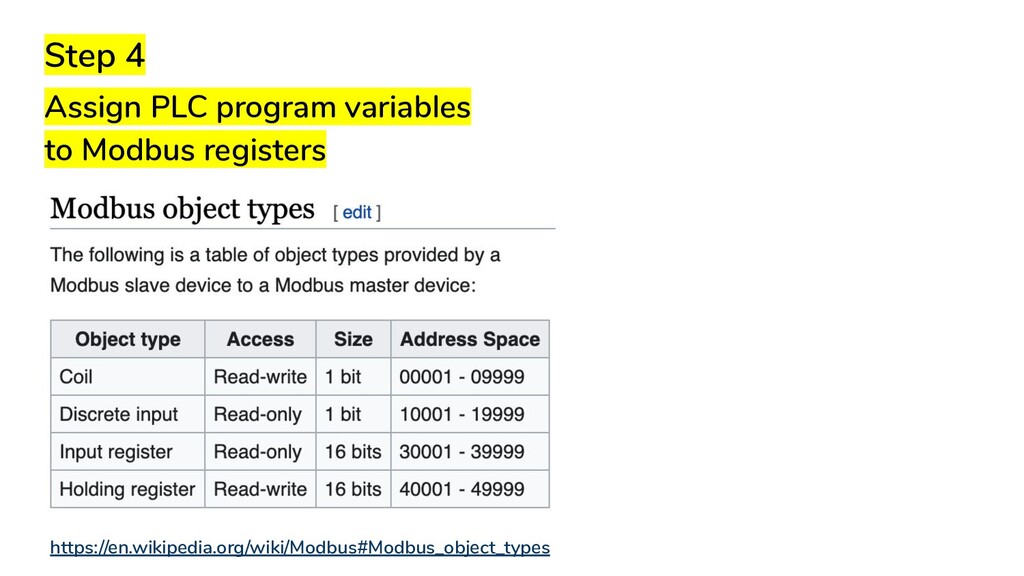
sleep ( 0.05 ) def get_current_tool_id (): return client. write_register ( 311, 1 ) # Wait for end of calibration while client. write_registers ( 620, int ( number_to_raw_data ( height_offset * 1000 ))) def auto_calibration (): print "Calibrate Robot if needed. write_registers ( 626, workspace_request_register ) def register_height_offset ( height_offset ): client. sleep ( 0.01 ) def register_workspace_name ( ws_name ): workspace_request_register = string_to_register ( ws_name ) client. write_registers ( 0, joint_to_send ) client. to_registers () return payload # - Modbus server related function def back_to_observation (): # To change # joint_real = joint_simu = joint_to_send = list ( map ( lambda j : int ( number_to_raw_data ( j * 1000 )), joint_simu )) client.
#Python modbus server code#
Those enums are the one used by the modbus server to receive requests class ColorEnum ( Enum ): ANY = - 1 BLUE = 1 RED = 2 GREEN = 3 NONE = 0 class ShapeEnum ( Enum ): ANY = - 1 CIRCLE = 1 SQUARE = 2 TRIANGLE = 3 NONE = 0 # Functions to convert variables for/from registers # Positive number : 0 - 32767 # Negative number : 32768 - 65535 def number_to_raw_data ( val ): if val > 15 ) = 1 : val = - ( val & 0x7FFF ) return val def string_to_register ( string ): # code a string to 16 bits hex value to store in register builder = BinaryPayloadBuilder () builder. #!/usr/bin/env python from import ModbusTcpClient from pymodbus.payload import BinaryPayloadBuilder, BinaryPayloadDecoder import time from enum import Enum, unique # Enums for shape and color.
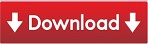